개발일지/Java
Java 상속 ( Inheritance )
E-room
2022. 9. 12. 11:55
728x90
기존의 클래스를 재활용하여 새로운 클래스를 작성하는 자바의 문법 요소
상속
두 클래스를 상위 클래스와 하위 클래스로 나누어 상위 클래스의 멤버(필드, 메서드. 이너 클래스)를 하위 클래스와 공유하는 것.
하위 클래스의 멤버 개수는 항상 상위 클래스와 같거나 많다.
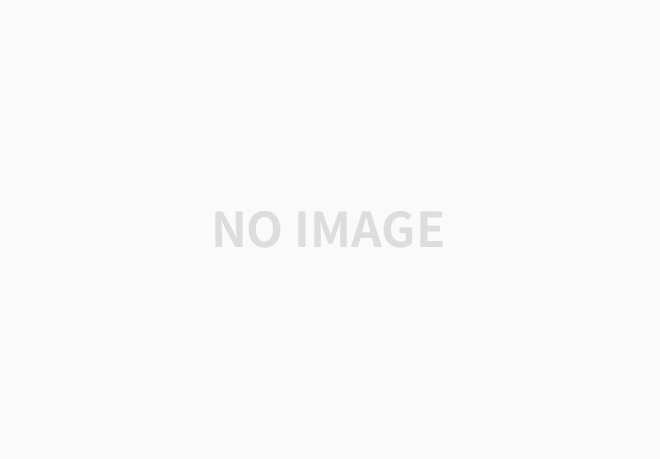
- 상위 클래스를 연장하여 만든 클래스에 새로운 기능을 추가하여 사용할 수 있다
- 상속을 통해 코드를 재사용하여 코드의 중복을 제거할 수 있다
- 다형적 표현이 가능
- 단일 상속만 가능 ( 여러 클래스를 상속받을 수 없음 )
class Person {
String name;
int age;
void learn(){
System.out.println("공부를 합니다.");
};
void walk(){
System.out.println("걷습니다.");
};
void eat(){
System.out.println("밥을 먹습니다.");
};
}
class Programmer extends Person { // Person 클래스로부터 상속. extends 키워드 사용
String companyName;
void coding(){
System.out.println("코딩을 합니다.");
};
}
public class Main {
public static void main(String[] args){
//Person 객체 생성
Person p = new Person();
p.name = "김코딩";
p.age = 24;
p.learn();
p.eat();
p.walk();
System.out.println(p.name);
//Programmer 객체 생성
Programmer pg = new Programmer();
pg.name = "박해커";
pg.age = 26;
pg.learn(); // Persons 클래스에서 상속받아 사용 가능
pg.coding(); // Programmer의 개별 기능
System.out.println(pg.name);
}
}
포함 관계
상속처럼 클래스를 재사용할 수 있는 방법으로, 클래스의 멤버로 다른 클래스 타입의 참조 변수를 선언하는 것
public class Employee {
int id;
String name;
Address address;
public Employee(int id, String name, Address address) {
this.id = id;
this.name = name;
this.address = address;
}
void showInfo() {
System.out.println(id + " " + name);
System.out.println(address.city+ " " + address.country);
}
public static void main(String[] args) {
Address address1 = new Address("서울", "한국");
Address address2 = new Address("도쿄", "일본");
Employee e = new Employee(1, "김코딩", address1);
Employee e2 = new Employee(2, "박해커", address2);
e.showInfo();
e2.showInfo();
}
}
class Address {
String city, country;
public Address(String city, String country) {
this.city = city;
this.country = country;
}
}
메서드 오버 라이딩 (Method Overriding)
상위 클래스로부터 상속받은 메서드와 동일한 이름의 메서드를 재정의하는 것 (덮어쓰는 것)
조건
- 메서드의 선언부(메서드 이름, 매개변수, 반환 타입)가 상위 클래스와 완전히 일치해야 함
- 접근 제어자의 범위가 상위 클래스의 메서드보다 같거나 넓어야 함
- 예외는 상위 클래스의 메서드보다 많이 선언 불가
장점
- 간편하게 배열로 선언하여 관리할 수 있음
public class Main {
public static void main(String[] args) {
Vehicle[] vehicles = new Vehicle[]{new Bike(), new Car(), new MotorBike()};
for (Vehicle vehicle : vehicles) {
vehicle.run();
}
}
}
class Vehicle {
void run() {
System.out.println("Vehicle is running");
}
}
class Bike extends Vehicle {
void run() {
System.out.println("Bike is running");
}
}
class Car extends Vehicle {
void run() {
System.out.println("Car is running");
}
}
class MotorBike extends Vehicle {
void run() {
System.out.println("MotorBike is running");
}
}
// 출력
Bike is running
Car is running
MotorBike is running
super, super()
this는 자신의 객체, this() 메서드는 자신의 생성자 호출이다.
super는 상위 클래스의 객체, super()는 상위 클래스의 생성자 호출이다.
super 키워드를 사용하여 부모 객체의 멤버 값을 참고할 수 있다.
public class Super {
public static void main(String[] args) {
Lower l = new Lower();
l.callNum();
}
}
class Upper {
int count = 20; // super.count
}
class Lower extends Upper {
int count = 15; // this.count
void callNum() {
System.out.println("count = " + count);
System.out.println("this.count = " + this.count);
System.out.println("super.count = " + super.count);
}
}
// 출력값
count = 15
count = 15
count = 20
super() 메서드 또한 this()와 마찬가지로 생성자 안에서만 사용 가능하고, 반드시 첫 줄에 와야 한다
public class Test {
public static void main(String[] args) {
Student s = new Student();
}
}
class Human {
Human() {
System.out.println("휴먼 클래스 생성자");
}
}
class Student extends Human { // Human 클래스로부터 상속
Student() {
super(); // Human 클래스의 생성자 호출
System.out.println("학생 클래스 생성자");
}
}
// 출력값
휴먼 클래스 생성자
학생 클래스 생성자
Object 클래스
자바의 모든 클래스는 Object 클래스로부터 확장된다
자바 컴파일러는 컴파일링 과정에서 다른 클래스로부터 아무런 상속을 받지 않는 클래스에 자동으로 extends Object를 추가한다
Object 클래스의 대표적인 메서드
메서드명 | 반환 타입 | 주요 내용 |
toString() | String | 객체 정보를 문자열로 출력 |
equals(Object obj) | boolean | 등가 비교 연산(==)과 동일하게 스택 메모리값을 비교 |
hashCode() | int | 객체의 위치정보 관련. Hashtable 또는 HashMap에서 동일 객체여부 판단 |
wait() | void | 현재 쓰레드 일시정지 |
notify() | void | 일시정지 중인 쓰레드 재동작 |
728x90